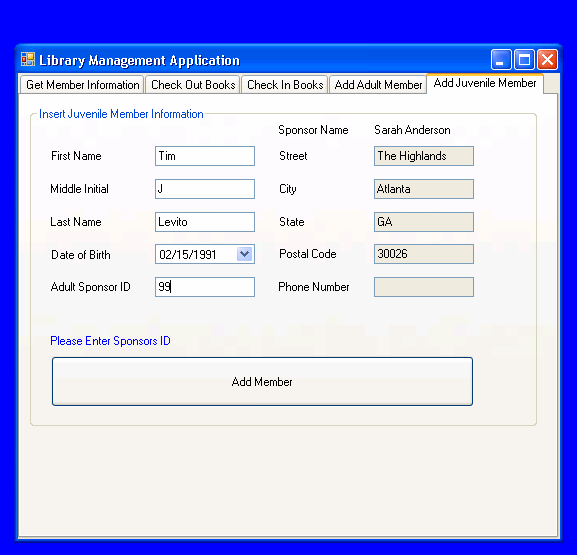
(Above)
Form level Validation takes a majority of the Format Checking workload and the user recieves easy to read and follow
messages to direct them to the proper information necessary to complete the transaction.
For Example, the form is asking for the Sponsor's ID value in order to add the Juvenile Member.
(Below)
Once the button is clicked, the form's button_click event is executed.
Here is the event's handler code in C#.
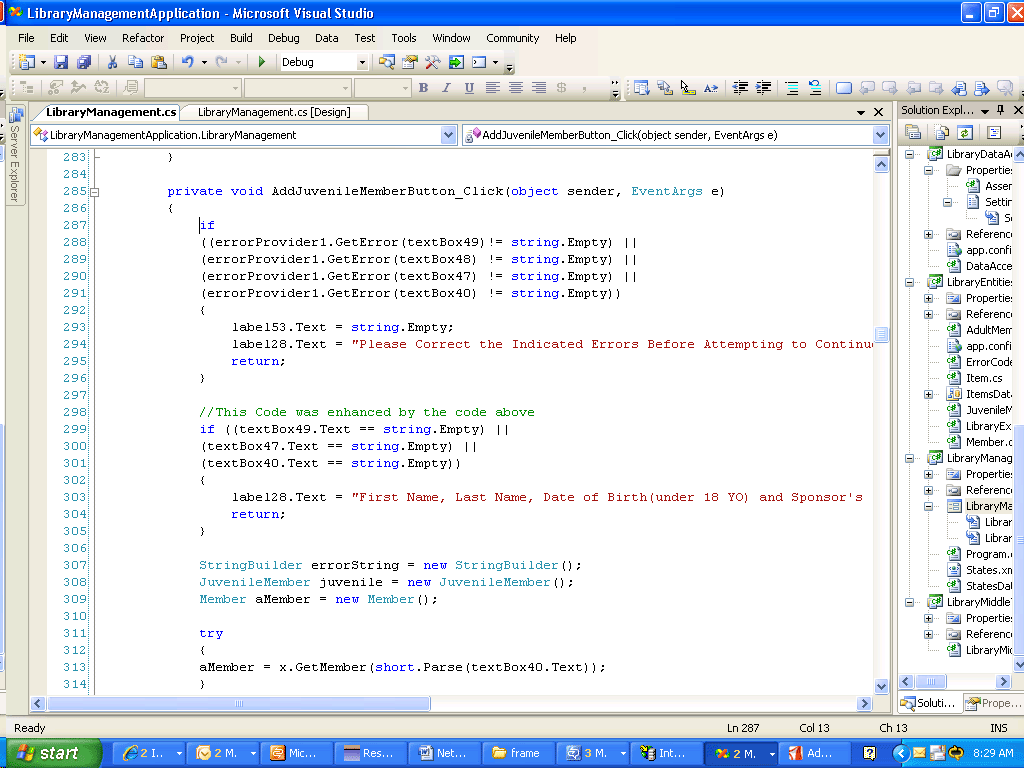
As with any good C# program, the first task is to double check your validation. Getting clean data at every level will
encourage and support a healthy database.
After the Validation, the Code behind the form's button_clicked event handler gets to the business of making the
call throught the .Net Layers to the waiting stored Procedures and eventually the database.
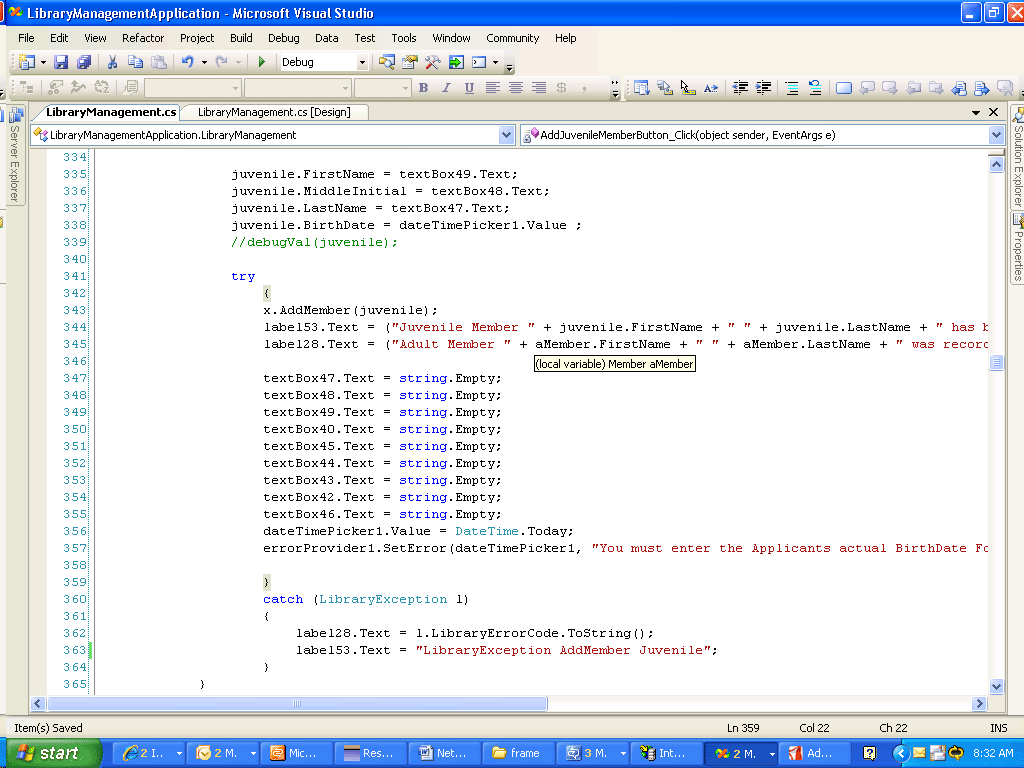
For clarification "x" is the instantiation of the LibraryMiddleTier class which in the framework design is the "Business
Layer". The call to
x.AddMember(juvenile);
calls the public method AddMember of the LibraryMiddleTier Class and passes a Juvenile Object as a parameter. The assigment
statements above load the properties of the Juvenile.
juvenile.firstName = textBox49.Text;
The Method call and reseting of the forms textboxs are all enclosed in a "Try Catch" block so that any errors can be
handled properly before propagating to the end user.
The call to the Business layer is the method shown below.
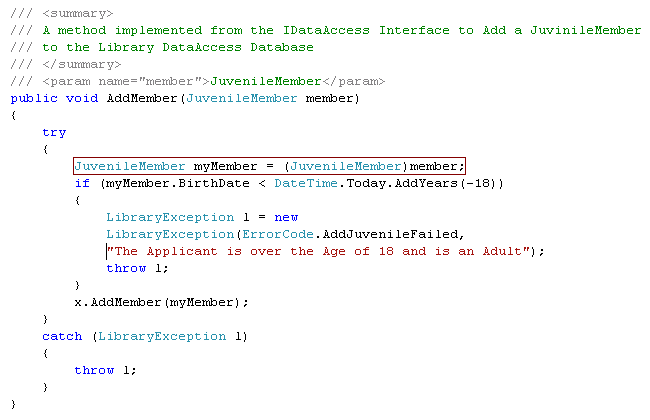
The validation on the data in the JuvenileMember object properties are performed in other methods in the Business Layer
and this point in the code have already been called from the forms button click event handler.
At this stage, the method tests a business rule before allowing the request to move closer to the database. A JuvenileMember
must be under the age of 18 in order to be a "Juvenile" member. Therefore the call to the DataAccess layer is enclosed in
a conditional "if" statement. Basically the condition reads, "If the juveniles birth date occurs after today's date minus
18 years, allow the transaction to proceed".
The Business layer is the best place for enforcing rules that are based on the operation of the business. In this simple
example, the Juvenile objects birth date is checked to make sure the member is under the age of 18 years
old before allowing them to be continue to the data access layer. If the age were changed to 19, this would be the
place in the application to change that business rule.
Once this business rule is satisfied, its time to make the call to the ADO.Net DataAccess Layer in the statement "x.AddMember(myMember);".
In this statement "x" is the instantiation of a DataAccess class object, "AddMember" is the Method in the object being called
and "(myMember)" Is the instantiated JuvenileMember object with it's properties set being passed again as a parameter.
Even thought the method signatures in the Business Layer and the Data Access Layer are essentially the same there is
no confusion when the compiler resolves at compile or runtime because they are contained in separate Namespaces.
At the DataAccess Layer, the connection to the Database is Created, and this "JuvenileMember" object's properties are
passed as separate parameters to the "TL_AddMember" Stored Procedure. The stored procedure is responsible for execution of
the group of related database requests (transaction) to add the juvenile member to the system.
In the next example, we will take a look at using Visual Studio 2005 and the types of code necessary to create
a Web interface to this same Library Management Application using ASP.Net, HTML, JavaScript, and the IIS Web Server.
|